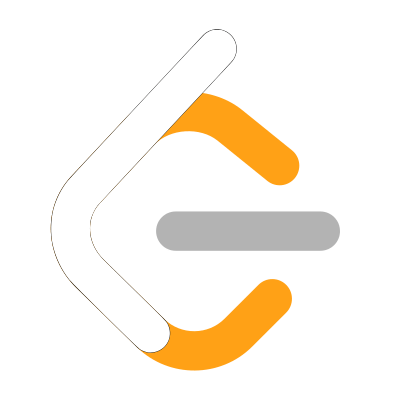
Two Sum solution using TypeScript
Below is my TypeScript solution to the LeetCode "Two Sum" question using a Hash Map to find the compliment.
Time Complexity: Because each element in the array will be visited one time, the time complexity is O(n), where n represents the number of elements in the array.
Space Complexity: This approach will place n elements in the map in the worst case, making the space complexity O(n).
/**
* Given an array of integers, return indices of the two numbers
* such that they add up to a specific target.
*
* Time Complexity: O(n)
* Space Complexity: O(n)
*
* Input: nums = [2, 7, 11, 15], target = 9
* Output: [0, 1]
* Because nums[0] + nums[1] = 2 + 7 = 9
*/
function twoSum(nums: number[], target: number): number[] {
const map: Map<number, number> = new Map();
let result: number[] = [];
for (let i = 0; i < nums.length; i++) {
const current = nums[i];
const match = map.get(target - current);
if (match !== undefined) {
result = [i, match];
break;
}
map.set(current, i);
}
return result;
}