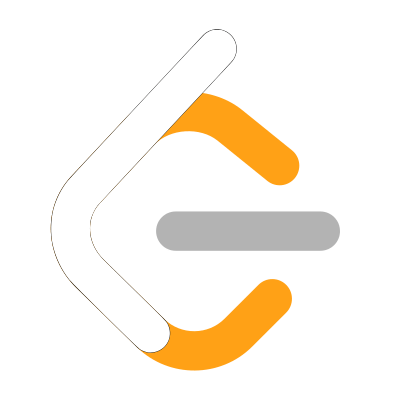
Min Stack solution using TypeScript
This post outlines my TypeScript solution to the "Min Stack" question on LeetCode.
Algorithm:
This solution uses a single stack. Each node on the stack contains two numbers: the value that was pushed, and the minimum value on the stack at the time of pushing the node onto the stack. This allows the class to provide a getMin
method that runs in constant time.
Time Complexity: Because all methods of the stack class consist only of operations that run in constant time, the time complexity is O(1).
Space Complexity:
Each method of the class uses a constant amount of space except for the push
method, which stores the element on the stack. This makes the space complexity O(n) where n represents the number of elements pushed onto the stack.
/**
* Min Stack
* Design a stack that supports push, pop,
* top, and retrieving the minimum element
* in constant time.
*/
class MinStack {
private stack: [number, number][] = []
constructor() {}
/**
* Push element x onto stack.
* Time Complexity: O(1);
* Space Complexity: O(n);
*/
push(x: number): void {
const min = this.stack.length
? Math.min(this.getMin(), x)
: x; // only number in stack
this.stack.push([x, min]);
}
/**
* Removes the element on top of the stack.
* Time Complexity: O(1);
* Space Complexity: O(1);
*/
pop(): void {
this.stack.pop();
}
/**
* Get the top element.
* Time Complexity: O(1);
* Space Complexity: O(1);
*/
top(): number {
return this.stack[this.stack.length - 1][0];
}
/**
* Retrieve the minimum element in the stack.
* Time Complexity: O(1);
* Space Complexity: O(1);
*/
getMin(): number {
return this.stack[this.stack.length - 1][1];
}
}