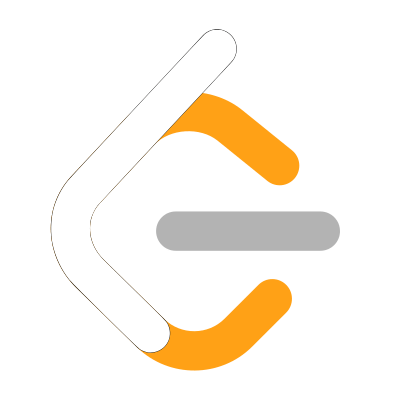
Fizz Buzz solution using TypeScript
This post outlines my TypeScript solution to the "Fizz Buzz" question on LeetCode.
Algorithm:
This solution creates a hash map of all values that should map to a word. In this case, only 3
and 5
. On each iteration, check each value in the hash table for divisibility. If the number is divisible by the key, concatenate the corresponding value to the string. Push the word to the array if a corresponding key way found, otherwise push the number. Finally, return the array.
Time Complexity: Because this solution will iterate n times, the time complexity is O(n) where n represents the inputted number.
Space Complexity: Because this solution will use a constant amount of space, the space complexity is O(1).
/**
* Fizz Buzz
* Write a program that outputs the string
* representation of numbers from 1 to n.
* But for multiples of three it should output
* “Fizz” instead of the number and for the multiples
* of five output “Buzz”. For numbers which are
* multiples of both three and five output “FizzBuzz”.
*
* Time Complexity: O(n)
* Space Complexity: O(1)
*/
function fizzBuzz(n: number): string[] {
const result: string[] = [];
const map = new Map([
[3, 'Fizz'],
[5, 'Buzz']
]);
let num = 1;
while (num <= n) {
let word = '';
map.forEach((val, key) => {
if (num % key === 0) {
word += val;
}
})
result.push(word || `${num}`);
num += 1;
}
return result;
}