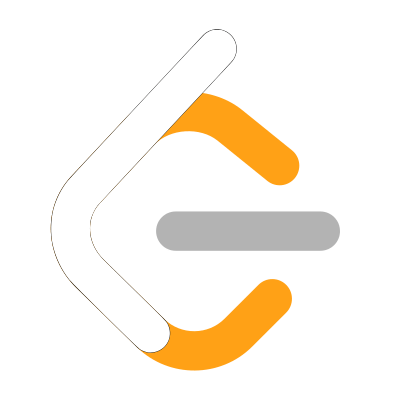
Delete Node in a Linked List solution using JavaScript
Below is my JavaScript solution to the LeetCode "Delete Node in a Linked List" question.
Time Complexity: Because this will only handle one node at a time, the time complexity is O(1).
Space Complexity: Because this approach will also only mutate the node that is passed in, the space complexity is O(1).
/**
* Definition for singly-linked list.
* function ListNode(val) {
* this.val = val;
* this.next = null;
* }
*
* Notes:
* - The linked list will have at least two elements.
* - All of the nodes' values will be unique.
* - Do not return anything from your function.
* - The given node will not be the tail and it will always
* be a valid node of the linked list.
*/
/**
* Delete a node (except the tail) in a singly linked
* list, given only access to that node.
*
* Time Complexity: O(1)
* Space Complexity: O(1)
*
* @param {ListNode} node
* @return {void}
*/
const deleteNode = node => {
node.val = node.next.val;
node.next = node.next.next;
};