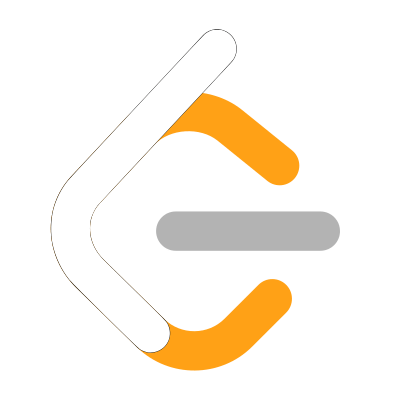
Roman to Integer solution using TypeScript
Below is my TypeScript solution to the LeetCode "Roman to Integer" question.
Time Complexity: Because each character in the string will be visited only one time, and the string is of a limited length, the time complexity is O(1). If there were not a limit on the length of the string, the approach would have a time complexity of O(n).
Space Complexity: This approach will also use a constant amount of space, so the space complexity is O(1).
/**
* Find the numerical value of a given string representing
* a Roman Numeral. For this exercise, Input is guaranteed
* to be within the range from 1 to 3999.
*
* Time Complexity: O(1)
* Space Complexity: O(1)
*
* Input: "MCMXCIV"
* Output: 1994
* Explanation: M = 1000, CM = 900, XC = 90 and IV = 4.
*/
function romanToInt(s: string): number {
const map = new Map([
['I', 1],
['IV', 4],
['V', 5],
['IX', 9],
['X', 10],
['XL', 40],
['L', 50],
['XC', 90],
['C', 100],
['CD', 400],
['D', 500],
['CM', 900],
['M', 1000]
]);
let result = 0;
for(let i = 0; i < s.length; i++) {
const twoSymbols = map.get(s[i] + s[i + 1]);
const oneSymbol = map.get(s[i]);
if (twoSymbols) {
i += 1; // skip iteration of next symbol
}
result += twoSymbols || oneSymbol || 0;
}
return result;
};