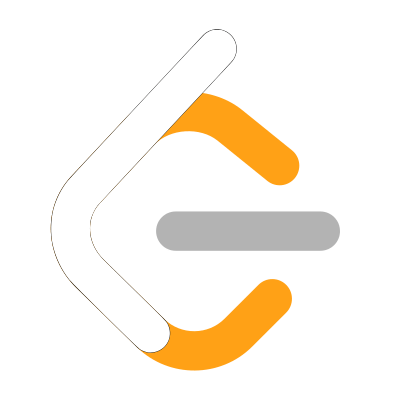
Remove Element solution using TypeScript
This post outlines my TypeScript solution to the "Remove Element" question on LeetCode.
Algorithm: The solution simply uses a pointer to iterate over the array. When the pointer finds a matching value, it splices it out of the array. When it reaches the end of the array, it returns the length of the array.
Time Complexity: Because the array will be iterated one time, the time complexity is O(n) where n represents the number of elements in the array.
Space Complexity: Because the solution will use a constant amount of space, the space complexity is O(1).
/**
* Remove Element
* Given an array nums and a value val, remove all
* instances of that value in-place and return the new length.
*
* Time Complexity: O(n)
* Space Complexity: O(1)
*
* removeElement([3,2,2,3], 3) // 3, [2,2]
* removeElement([0,1,2,2,3,0,4,2], 2) // 2, [0,1,3,0,4]
*/
function removeElement(nums: number[], val: number): number {
let ptr = 0;
while (ptr < nums.length) {
if (nums[ptr] === val) {
nums.splice(ptr, 1);
continue;
}
ptr += 1;
}
return nums.length;
}