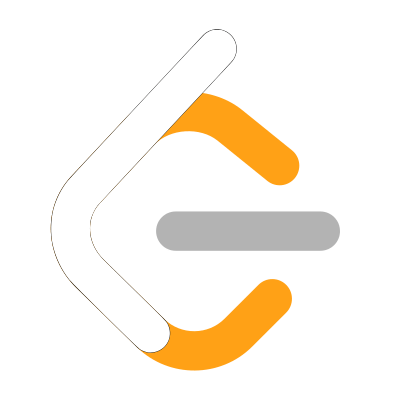
Merge Two Sorted Lists solution using TypeScript
Below is my TypeScript solution to the LeetCode "Merge Two Sorted Lists" question using a recursive approach.
Time Complexity: Because each node in each of the linked lists will be visited one time, the time complexity is O(m + n), where m represents the number of nodes in the first linked list and n represents the number of nodes in the second linked list.
Space Complexity: This approach will place m + n function calls on the stack, making the space complexity O(m + n).
/**
* Definition for singly-linked list.
* class ListNode {
* val: number
* next: ListNode | null
* constructor(val?: number, next?: ListNode | null) {
* this.val = (val===undefined ? 0 : val)
* this.next = (next===undefined ? null : next)
* }
* }
*/
/**
* Merge two sorted linked lists and return it as a new sorted list.
*
* Time Complexity: O(m + n)
* Space Complexity: O(m + n)
*
* Input: 1->2->4, 1->3->4
* Output: 1->1->2->3->4->4
*/
function mergeTwoLists(l1: ListNode | null, l2: ListNode | null): ListNode | null {
if (l1 === null) {
return l2;
}
else if (l2 === null) {
return l1;
}
else if (l1.val < l2.val) {
l1.next = mergeTwoLists(l1.next, l2);
return l1;
} else {
l2.next = mergeTwoLists(l1, l2.next);
return l2
}
}