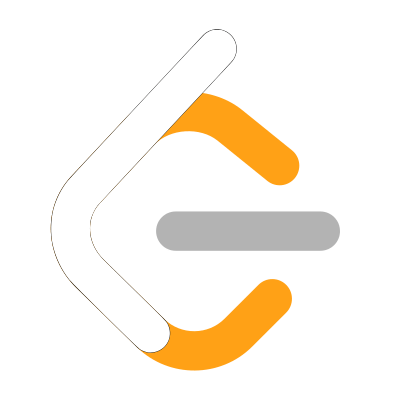
Length of Last Word solution using TypeScript
Below is my TypeScript solution to the LeetCode "Length of Last Word" question.
Time Complexity: Because each character in the string will be visited, at most, only one time, the time complexity is O(n), where n represents the number of characters in the string.
Space Complexity: This approach will also use a constant amount of space, making the space complexity O(1).
/**
* Given a string s consisting of upper/lower-case alphabets and
* empty space characters ' ', return the length of last
* word in the string.
*
* Time Complexity: O(n)
* Space Complexity: O(1)
*
* Input: "Test input"
* Output: 5
*
* Input: "Ignore spaces at end "
* Output: 3
*
* Input: "NoSpaces"
* Output: 8
*/
function lengthOfLastWord(s: string): number {
// place a pointer at the end of the string
let ptr = s.length;
let length = 0;
while (ptr > 0) {
ptr -= 1;
// currently iterating through the last word
if (s[ptr] !== ' ') {
length += 1;
}
// boundary of the last word has been reached
else if (length > 0) {
break;
}
}
return length;
};