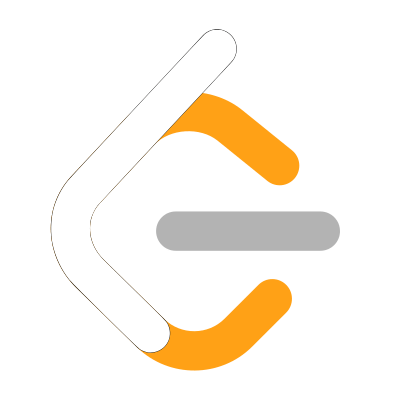
Convert a Number to Hexadecimal solution using TypeScript
This post outlines my TypeScript solution to the LeetCode "Convert a Number to Hexadecimal" question using an iterative bitwise solution.
Algorithm: The solution iterates over the base 10 input to find a hexadecimal representation of the number by doing the following:
- Create a map of decimal digits to hexadecimal digits. This can be represented by a string of every hexadecimal digit.
- Take the last 4 binary digits (a nibble) of the input, use its decimal value as an index of the created map to retrieve its hexadecimal value. Prepend the hexadecimal value to returned string.
- Shift the input 4 binary digits to the right.
- Continue taking and shifting binary digits (the above 2 steps) until the input is 0.
- Return the hexadecimal string.
Time Complexity: Because the input gets divided by 16 on every iteration, the time complexity is O(log(n)) where n represents the size of the input.
Space Complexity: Because the stored hex value is the remainder of dividing the input by 16 on every iteration, the space complexity is also O(log(n)) where n represents the size of the input.
/**
* Convert a Number to Hexadecimal
* Given an integer, write an algorithm to
* convert it to hexadecimal. For negative
* integer, two’s complement method is used.
*
* Time Complexity: O(log(n))
* Space Complexity: O(log(n))
*
* toHex(26) // "1a"
* toHex(4) // "4"
* toHex(-1) // "ffffffff"
*/
function toHex(num: number): string {
const map = "0123456789abcdef";
let hex = num === 0 ? "0" : "";
while (num !== 0) {
hex = map[num & 15] + hex;
num = num >>> 4;
}
return hex;
}