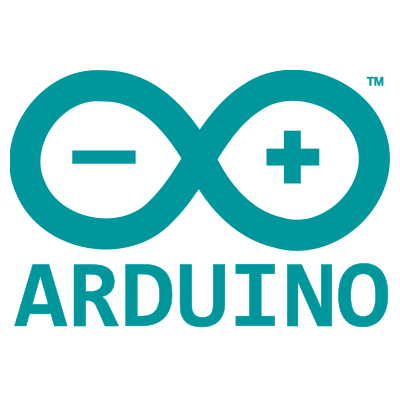
Simple hit counter sketch for Arduino Uno
A simple sketch I wrote up for the Arduino Uno. Every click of the switch will increment a hit counter and output it to the LCD.
// include the library code:
#include <LiquidCrystal.h>
// initialize the library with the numbers of the interface pins
LiquidCrystal lcd(12, 11, 5, 4, 3, 2);
// set up a constant for the tilt switchPin
const int switchPin = 6;
int hits = 0;
// variable to hold the value of the switchPin
int switchState = 0;
// variable to hold previous value of the switchpin
int prevSwitchState = 0;
void setup() {
// set up the number of columns and rows on the LCD
lcd.begin(16, 2);
// set up the switch pin as an input
pinMode(switchPin,INPUT);
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Hit the button");
lcd.setCursor(0, 1);
lcd.print("to increment");
}
void loop() {
// check the status of the switch
switchState = digitalRead(switchPin);
// compare the switchState to its previous state
if (switchState != prevSwitchState) {
if (switchState == LOW) {
hits = hits + 1;
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Hits:");
lcd.setCursor(0, 1);
lcd.print(hits);
}
}
// save the current switch state as the last state
prevSwitchState = switchState;
}