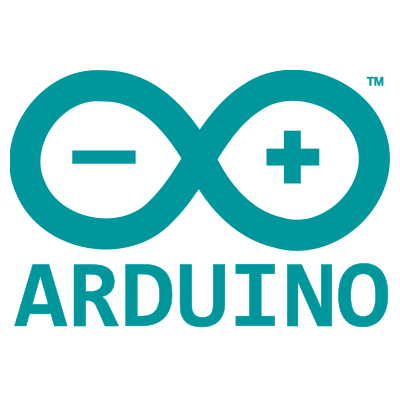
Simple flashing LEDs sketch for the Arduino UNO
The sketch below is a slightly modified version of the spaceship interface example. Pressing the button will cause the series of lights to blink rapidly.
// Create a global variable to hold the
// state of the switch. This variable is persistent
// throughout the program. Whenever you refer to
// switchState, you’re talking about the number it holds
int switchstate = 0;
void setup(){
// declare the LED pins as outputs
pinMode(3, OUTPUT);
pinMode(4, OUTPUT);
pinMode(5, OUTPUT);
// declare the switch pin as an input
pinMode(2, INPUT);
}
void loop(){
// read the value of the switch
// digitalRead() checks to see if there is voltage
// on the pin or not
switchstate = digitalRead(2);
// if the button is not pressed, turn on only the bottom LED
if (switchstate == LOW) {
digitalWrite(3, HIGH);
digitalWrite(4, LOW);
digitalWrite(5, LOW);
}
// if the button is pressed, flash all LEDs rapidly
else {
digitalWrite(3, HIGH);
digitalWrite(4, LOW);
digitalWrite(5, LOW);
delay(50);
digitalWrite(3, LOW);
digitalWrite(4, HIGH);
digitalWrite(5, LOW);
delay(50);
digitalWrite(3, LOW);
digitalWrite(4, LOW);
digitalWrite(5, HIGH);
delay(50);
}
}