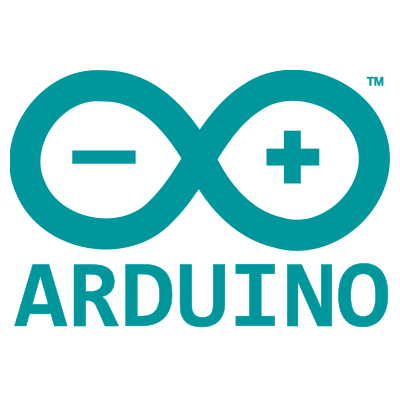
Firmware Loading via bluetooth for the Arduino Uno
A while back I wrote the following script to load firmware onto the Arduino Uno via bluetooth. When I have a chance to dig up the wiring diagrams I'll add them to this post.
#include <LiquidCrystal.h>
int resetPin = 6;
LiquidCrystal lcd(12, 13, 11, 10, 9, 8);
void setup() {
Serial.begin(115200);
pinMode(resetPin, OUTPUT);
digitalWrite(resetPin, LOW);
// setup the number of columns and rows on the LCD
lcd.begin(16, 2);
lcd.clear();
}
void loop() {
// Check if avrdude 5.11 is attempting to push new firmware to the device
Serial.peek() == '0' ? downloadFirmware() : mainProgram();
delay(100);
}
void mainProgram() {
display("Listening for", "serial input :)");
}
void downloadFirmware() {
for(int i=0; i<100; i++) {
// wait up to 5 seconds for synchronization request from avrdude 5.11
if(Serial.read() == '0' && Serial.read()==' ') {
display("Updating", "firmware...");
// send response to avrdude 5.11 to begin synchronization
Serial.write(0x14);
Serial.write(0x10);
resetArduino();
}
delay(50);
}
}
void display(char *line1, char *line2) {
lcd.clear();
lcd.setCursor(0, 0);
lcd.print(line1);
lcd.setCursor(0, 1);
lcd.print(line2);
}
void resetArduino() {
digitalWrite(resetPin, HIGH);
}