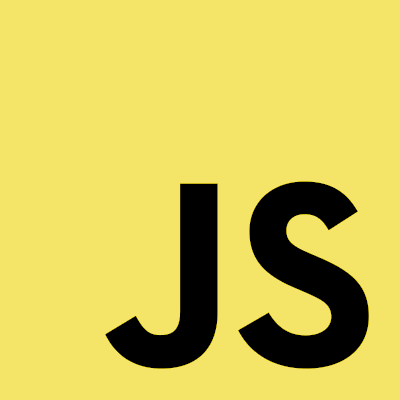
How to create a simple class in Javascript (ES5)
Below is an example of how to create a very basic class in Javascript using a function with methods added to the prototype.
var Basic = (function () {
function Basic(val) {
this.val = val;
}
Basic.prototype.output = function () {
return "Stored value is: " + this.val;
};
return Basic;
})();
var basic = new Basic("someValue");
console.log(basic.output());
By adding a method to the prototype, we avoid having to recreate the output
method every single time we create a new Basic
object.