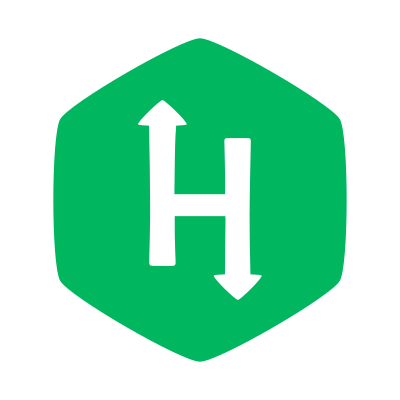
Plus Minus solution using JavaScript
Below is my JavaScript solution to the Plus Minus challenge on HackerRank.
Time Complexity: Because each element in the array will be visited only one time, the time complexity is O(n), where n represents the number of elements in the array.
Space Complexity: This approach will use a constant amount of space, making the space complexity O(1).
/**
* Given an array of numbers, calculate the fractions of its
* elements that are positive, negative, and are zeros.
* Print the decimal value of each fraction on a new line.
*
* Time Complexity: O(n)
* Space Complexity: O(1)
*
* Input: [-4, 3, -9, 0, 4, 1]
* Output:
* 0.500000
* 0.333333
* 0.166667
* Explanation:
* 3/6 numbers are positive
* 2/6 numbers are negative
* 1/6 numbers are zero
*/
function plusMinus(arr) {
const {positive, negative, zero} =
arr.reduce((acc, val) => {
if (val > 0) {
acc.positive += 1;
}
if (val === 0) {
acc.zero += 1;
}
if (val < 0) {
acc.negative += 1;
}
return acc;
}, {
positive: 0,
negative: 0,
zero: 0
});
console.log(positive / arr.length);
console.log(negative / arr.length);
console.log(zero / arr.length);
}